I have Ruby v2.6.7 installed using rvm and I’ll use Homebrew to install Node and Yarn on my Mac.
Installing software tends to either go swimmingly or you end up drowning in a Pool of Despair. In the Pool of Despair case, extra instructions don’t seem to help very much so I’ll keep mine to a minimum.
gem install rails # I'm on rails 6.1
brew install node # javascript runtime
brew install yarn # javascript package manager
Yarn is the equivalent of rubygems in the Javascript ecosystem. We’ll use yarn to install node packages.
Open a terminal and go to that favourite part of your hard drive where you keep all your future dreams and create a rails project.
rails new blogging --database=postgresql --webpack=react --skip-turbolinks --skip-spring
Go make a cup of tea while that is installing.
I use PostgresSQL because it makes deploying to Heroku easier. Use SQLite or MySQL if you prefer.
I don’t like Turbolinks.
On four Mac Books in a row, Spring always pegs my CPU at 100% and I don’t know why.
–webpack=react will install Webpack and the node packages for React.
Webpack replaces Sprockets for processing Javascript assets but we’ll still use Sprockets for CSS because I haven’t figured out to use Webpack for CSS yet and everyone says it’s complicated.
I’m going to push everything to Github so I can link to the code online. I just created a repository at https://github.com/klawrence/blogging
cd blogging
git init
git add .
git commit -m 'Start blogging!'
git remote add origin https://klawrence@github.com/klawrence/blogging.git
git push -u origin master
If the install gods are on our side we can create the database, start the server and see the happy, happy Welcome to Rails banner.
rails db:create rails server # Then in a new terminal open http://localhost:3000/
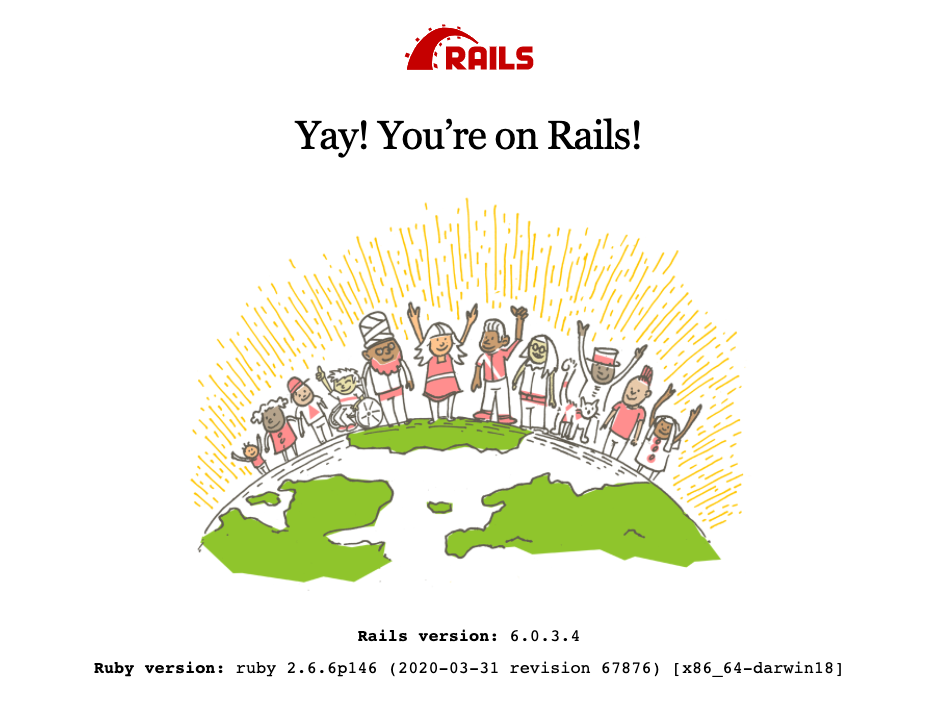
When we created our Rails project, Webpacker created a HelloReact app. We can use that to test that React installed correctly. We’ll create a posts controller to initialize HelloReact and we’ll set it as the home page in routes.rb.
rails generate controller Posts index
#routes.rb Rails.application.routes.draw do root to: 'posts#index' resources :posts end
Webpacker compiles javascript modules into packs and the javascript_pack_tag method will include your pack on a page. We’ll call it from the index page.
# views/posts/index.html.erb <%= javascript_pack_tag 'hello_react' %>
Webpacker ships with a utility that rebuilds your packs and refreshes the page whenever you change any Javascript. We’ll run it in a terminal window.
bin/webpack-dev-server
Now, when I go to http://localhost:3000/, I see the message “Hello, React”. I hope you do too.
Everything is set up now and we’re ready to start coding. In the next episode, we’ll show our first blog post.